Checking if a File Exists in Node.js: A Comprehensive Guide
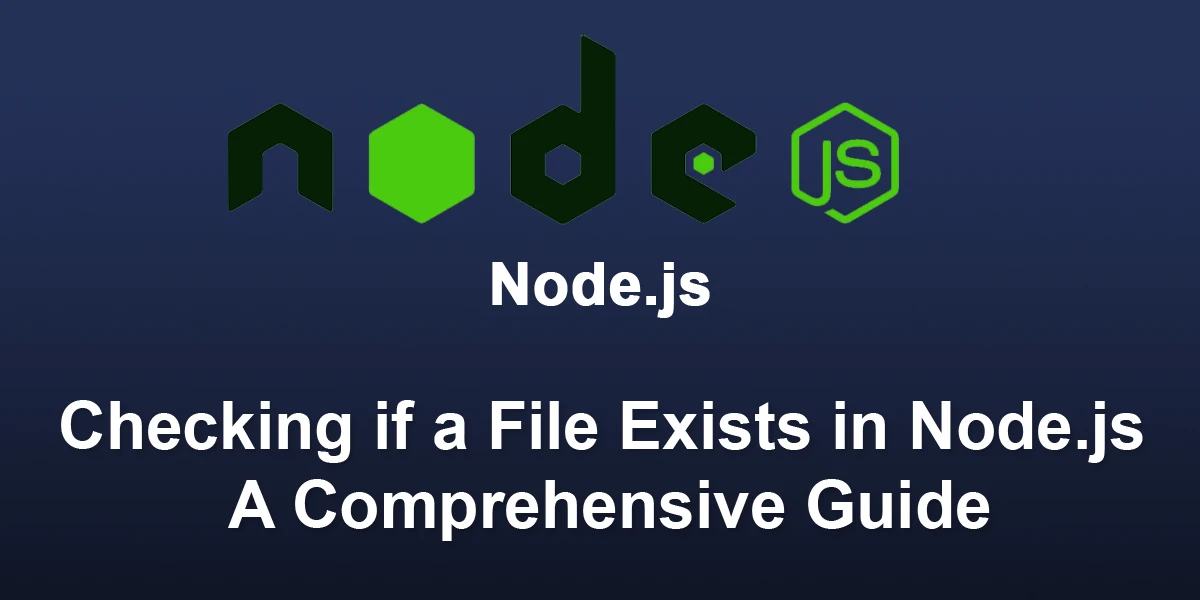
When working with file operations in Node.js, it’s crucial to check if a file exists before performing any read or write operations to avoid errors. In this article, we’ll explore various methods to check if a file exists in Node.js, providing you with a solid foundation for handling file existence in your applications.
Method 1: Using ‘fs.access’
The fs.access method is a versatile way to check if a file exists. It provides a low-level interface to file access permissions.
const fs = require('fs'); function checkFileExists(filePath) { fs.access(filePath, fs.constants.F_OK, (err) => { if (err) { console.error(`${filePath} does not exist`); } else { console.log(`${filePath} exists`); } }); } // Example usage const filePath = 'path/to/your/file.txt'; checkFileExists(filePath);
In this example, the fs.access method is used with the fs.constants.F_OK flag to check if the file exists. The callback function is called with an error if the file does not exist.
Method 2: Using ‘fs.stat’
The fs.stat method provides detailed information about a file, including whether it exists.
const fs = require('fs'); function checkFileExists(filePath) { fs.stat(filePath, (err, stats) => { if (err) { console.error(`${filePath} does not exist`); } else { console.log(`${filePath} exists`); } }); } // Example usage const filePath = 'path/to/your/file.txt'; checkFileExists(filePath);
The fs.stat method returns a stats object in the callback. If an error occurs, the file does not exist.
Method 3: Using ‘fs.promises.access’ (Node.js 12.0.0 and later)
For those using a Node.js version that supports promises, you can use fs.promises.access for a more streamlined approach.
const fs = require('fs').promises; async function checkFileExists(filePath) { try { await fs.access(filePath, fs.constants.F_OK); console.log(`${filePath} exists`); } catch (err) { console.error(`${filePath} does not exist`); } } // Example usage const filePath = 'path/to/your/file.txt'; checkFileExists(filePath);
Here, the fs.promises.access method is used with async/await syntax for better readability.
Method 4: Using ‘fs.existsSync’
While considered deprecated, fs.existsSync is still widely used for synchronous file existence checks.
const fs = require('fs'); function checkFileExists(filePath) { if (fs.existsSync(filePath)) { console.log(`${filePath} exists`); } else { console.error(`${filePath} does not exist`); } } // Example usage const filePath = 'path/to/your/file.txt'; checkFileExists(filePath);
Keep in mind that using fs.existsSync is synchronous, and for performance reasons, it’s recommended to use asynchronous methods in most cases.
You’ve learned multiple ways to check if a file exists in Node.js. Choose the method that best fits your application’s needs and coding style. Incorporate these checks into your file handling logic to ensure robust and error-free operations.