Error: Connection Lost in Node.js with MySQL
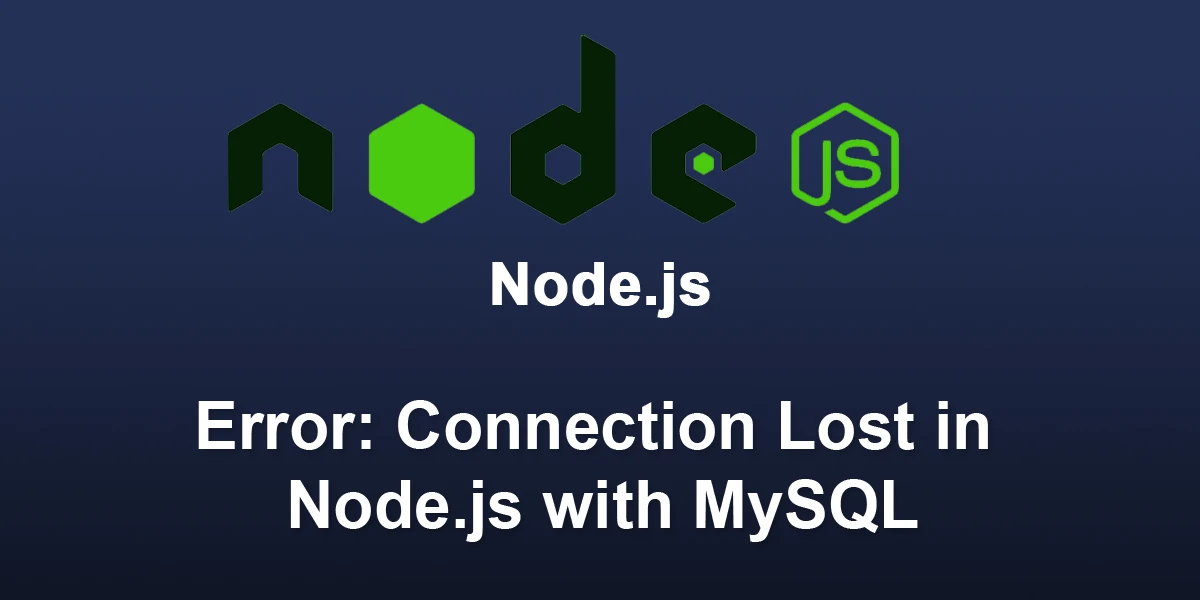
When working with Node.js and MySQL, encountering the “Error: Connection lost The server closed the connection” message is not uncommon. This error usually indicates a problem with the MySQL server or a timeout issue. In this article, we’ll explore common causes for this error and how to handle it in your Node.js application.
Understanding the Error
The “Error: Connection lost” error often occurs when there’s a long period of inactivity between the Node.js application and the MySQL server. MySQL connections have a timeout, and if a connection is idle for too long, the server may close it.
Handling the Error in Node.js
To handle this error, you can implement a connection pool and utilize the reconnect option provided by the mysql package. This ensures that if a connection is lost, the pool will attempt to reconnect automatically.
Let’s create a simple Node.js script to demonstrate handling this error:
// Install the mysql package using: npm install mysql const mysql = require('mysql'); // Create a connection pool const pool = mysql.createPool({ connectionLimit: 10, host: 'localhost', user: 'your_username', password: 'your_password', database: 'your_database', reconnect: true, // Enable automatic reconnection }); // Function to perform a sample query function querySample() { pool.getConnection((err, connection) => { if (err) throw err; // Perform a sample query connection.query('SELECT 1 + 1 AS solution', (error, results, fields) => { connection.release(); // Release the connection to the pool if (error) throw error; console.log('The solution is: ', results[0].solution); }); }); } // Execute the sample query every 10 seconds setInterval(querySample, 10000);
In this example:
- We create a connection pool using mysql.createPool.
- The reconnect option is set to true to enable automatic reconnection.
- We define a function querySample that performs a sample query and releases the connection back to the pool.
- The setInterval function is used to execute the querySample function every 10 seconds.
This script continuously performs a sample query, and if the connection is lost, the pool will attempt to reconnect automatically.
Handling the “Error: Connection lost” in Node.js with MySQL involves setting up a connection pool and enabling the reconnect option. This ensures that your application can gracefully handle lost connections and reconnect when necessary.
Note: Remember to replace the placeholder values in the connection configuration with your actual MySQL server details.