How to redirect to another page with angular route step by step
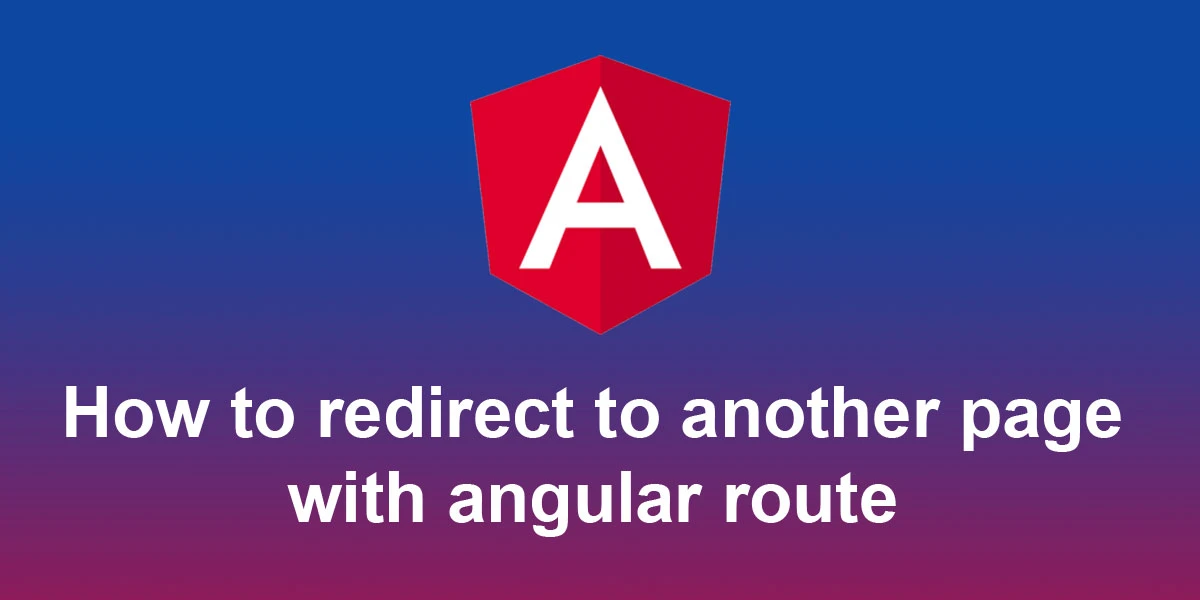
Here’s a step-by-step guide on how to redirect to another page using Angular’s router:
Step 1: Install the Angular Router
If you haven’t already installed the Angular Router, you can do so using the following command in your terminal:
ng add @angular/router
Step 2: Configure Routes
Open your ‘app-routing.module.ts’ file and configure your routes. For example:
// app-routing.module.ts import { NgModule } from '@angular/core'; import { RouterModule, Routes } from '@angular/router'; import { HomeComponent } from './home/home.component'; import { OtherComponent } from './other/other.component'; const routes: Routes = [ { path: 'home', component: HomeComponent }, { path: 'other', component: OtherComponent }, { path: '', redirectTo: '/home', pathMatch: 'full' }, ]; @NgModule({ imports: [RouterModule.forRoot(routes)], exports: [RouterModule], }) export class AppRoutingModule {}
Here, we’ve defined two routes: ‘home’ and ‘other’. The empty path ‘””‘ with ‘redirectTo’ is set to redirect to the ‘home’ route by default.
Step 3: Create Components
Make sure you have the components (HomeComponent and OtherComponent) that correspond to your routes.
Step 4: Use Router in a Component
In the component where you want to trigger the redirection (for example, ‘some.component.ts’), import the ‘Router’ and use the ‘navigate’ method:
// some.component.ts import { Component } from '@angular/core'; import { Router } from '@angular/router'; @Component({ selector: 'app-some', template: ``, }) export class SomeComponent { constructor(private router: Router) {} redirectToOtherPage() { this.router.navigate(['/other']); } }
This example assumes that you have a button in your component, and when it’s clicked, it triggers the ‘redirectToOtherPage’ method, which uses the ‘Router’ to ‘navigate’ to the ‘other’ route.
Step 5: Add Router Outlet in App Component
Ensure that your ‘app.component.html’ (or wherever your main component template is) contains a ‘
With these steps, you should be able to redirect to another page using Angular’s router. Adjust component and route names based on your actual application structure.