How to use angular multi select dropdown step by step
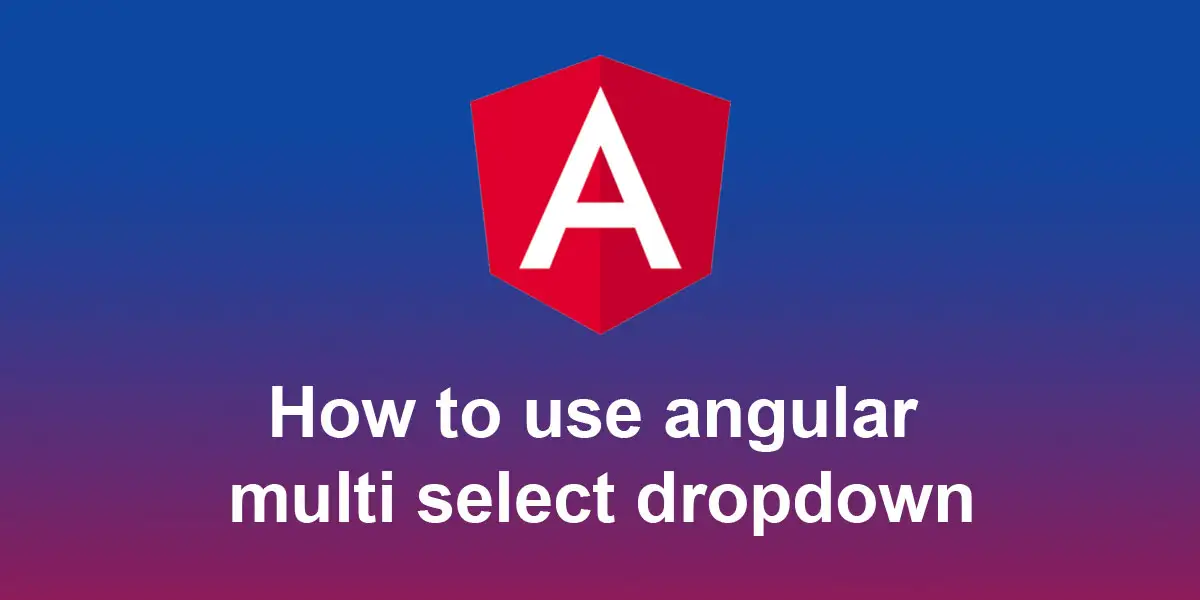
In Angular, you can create a multi-select dropdown using various libraries or by implementing a custom solution. One popular library for this purpose is ‘ng-multiselect-dropdown’. Here’s a step-by-step guide on how to use it:
Step 1: Install the library
Install the ‘ng-multiselect-dropdown’ library using npm:
npm install ng-multiselect-dropdown
Step 2: Import the module
Import the ‘NgMultiSelectDropDownModule’ in your Angular module (typically ‘app.module.ts’):
// app.module.ts import { BrowserModule } from '@angular/platform-browser'; import { NgModule } from '@angular/core'; import { NgMultiSelectDropDownModule } from 'ng-multiselect-dropdown'; import { AppComponent } from './app.component'; @NgModule({ declarations: [ AppComponent ], imports: [ BrowserModule, NgMultiSelectDropDownModule.forRoot() ], providers: [], bootstrap: [AppComponent] }) export class AppModule { }
Step 3: Use the component in your template
Use the ‘ng-multiselect-dropdown’ component in your template. For example, if you want to create a multi-select dropdown for a list of items:
Step 4: Define your data and settings in the component
In your component (e.g., ‘app.component.ts’), define the list of items, selected items, and dropdown settings:
// app.component.ts import { Component } from '@angular/core'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { dropdownList = []; selectedItems = []; dropdownSettings = {}; constructor() { this.dropdownList = [ { item_id: 1, item_text: 'Item 1' }, { item_id: 2, item_text: 'Item 2' }, // Add more items as needed ]; this.selectedItems = [ // Set default selected items if needed ]; this.dropdownSettings = { singleSelection: false, idField: 'item_id', textField: 'item_text', selectAllText: 'Select All', unSelectAllText: 'Unselect All', allowSearchFilter: true }; } }
Make sure to adjust the data and settings based on your specific requirements.
Step 5: Styling (Optional)
You can apply custom styles to the dropdown by using CSS. Customize the styles in your component’s CSS file.
Step 6: Run your Angular application
Run your Angular application using the ng serve command and navigate to the appropriate URL in your browser. You should see the multi-select dropdown in action.
Remember to check the documentation of ng-multiselect-dropdown for any additional features or customization options: ng-multiselect-dropdown GitHub