Create React contact form and send email with EmailJS
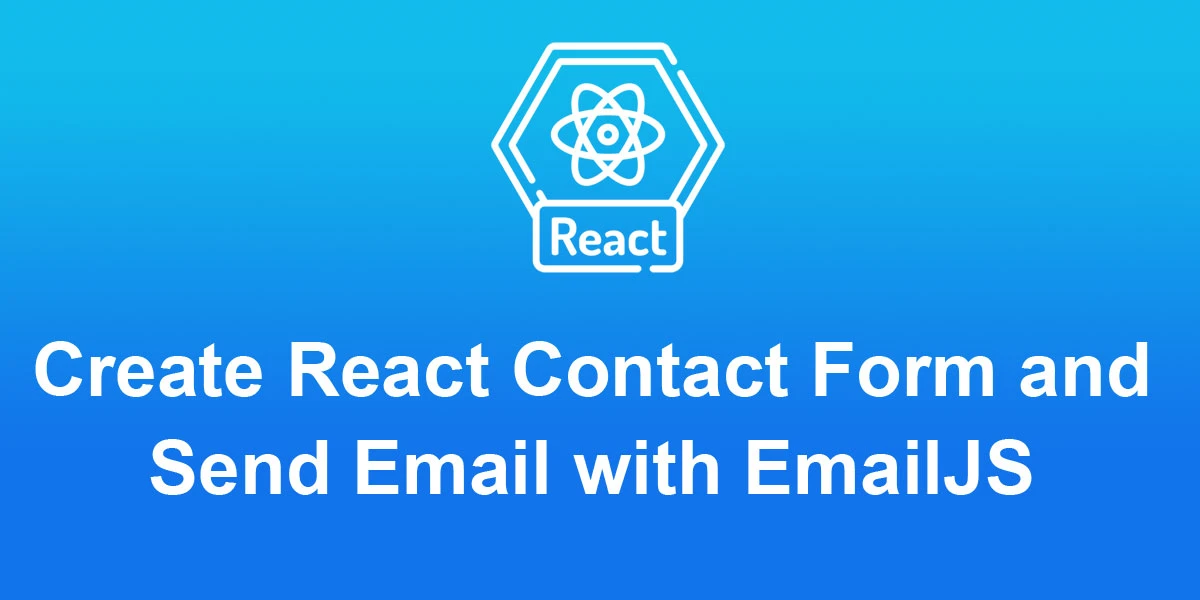
Creating a contact form with EmailJS in a React application involves a few steps. EmailJS is a service that allows you to send emails directly from the client-side using JavaScript. Here’s a step-by-step guide:
Step 1: Set Up Your React App
Assuming you have a React app already set up, if not, you can create one using:
npx create-react-app my-emailjs-app cd my-emailjs-app
Step 2: Install EmailJS Library
Install the EmailJS library using npm or yarn:
npm install emailjs-com
or
yarn add emailjs-com
Step 3: Set Up an EmailJS Account
Go to EmailJS and sign up for an account. Once logged in, create a new email service and template.
Step 4: Get Your EmailJS Service ID and Template ID
After creating a service and template, you’ll need to get the Service ID and Template ID. You can find these in the EmailJS dashboard under the “Email Services” and “Email Templates” sections.
Step 5: Create a Contact Form Component
Create a new component for your contact form, for example, ‘ContactForm.js’.
// ContactForm.js import React, { useState } from 'react'; import emailjs from 'emailjs-com'; const ContactForm = () => { const [name, setName] = useState(''); const [email, setEmail] = useState(''); const [message, setMessage] = useState(''); const handleSubmit = (e) => { e.preventDefault(); // EmailJS parameters const templateParams = { from_name: name, from_email: email, message, }; // EmailJS API call emailjs .send( 'YOUR_SERVICE_ID', // Replace with your EmailJS Service ID 'YOUR_TEMPLATE_ID', // Replace with your EmailJS Template ID templateParams, 'YOUR_USER_ID' // Replace with your EmailJS User ID ) .then( (response) => { console.log('Email sent successfully:', response); }, (error) => { console.error('Email send failed:', error); } ); }; return (); }; export default ContactForm;
Step 6: Use the Contact Form in Your App
Import and use the ‘ContactForm’ component in your main app file (‘App.js’ or similar).
// App.js import React from 'react'; import ContactForm from './ContactForm'; const App = () => { return (); }; export default App;Contact Us
Step 7: Replace EmailJS Credentials
Replace the placeholder values in the ‘send’ function with your actual EmailJS Service ID, Template ID, and User ID.
Now, when users submit the form, it will send an email using EmailJS. Remember not to expose sensitive information like User IDs in your client-side code in production.
This is a basic setup, and you can customize the form and handling as per your application’s requirements.