How to API call in ReactJS step by step guide
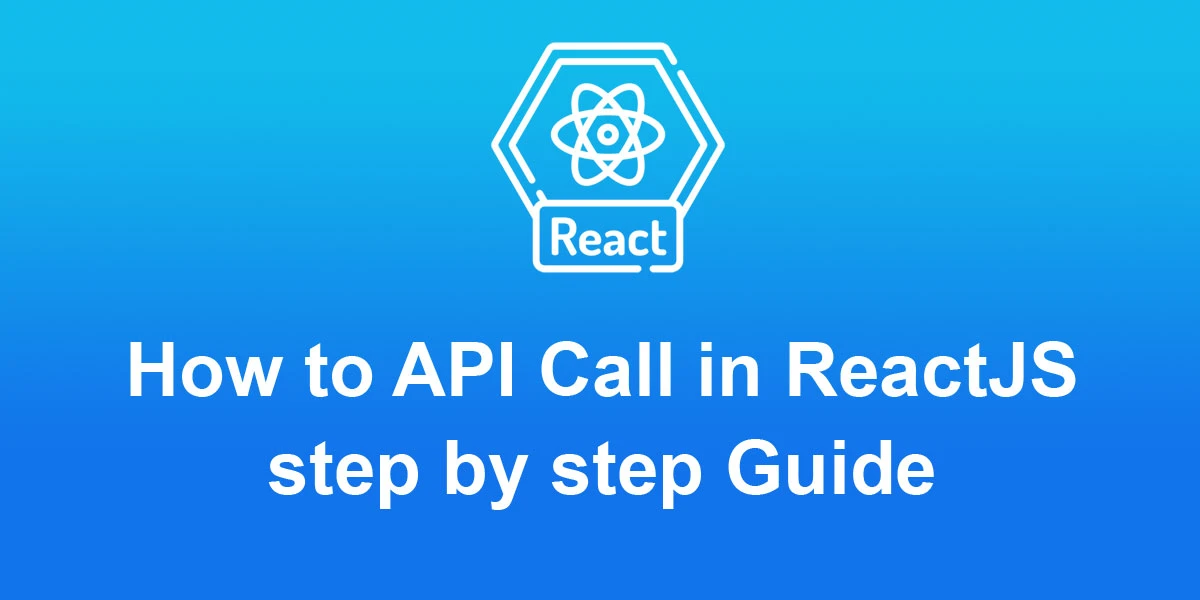
Calling an API in a React application involves using the fetch function or libraries like axios. Here’s a step-by-step guide using the fetch function:
Step 1: Set Up Your React App
Assuming you have a React app already set up. If not, you can create one using:
npx create-react-app my-api-app cd my-api-app
Step 2: Create a Component for API Call
Create a new component, for example, ‘ApiComponent.js’.
// src/components/ApiComponent.js import React, { useEffect, useState } from 'react'; const ApiComponent = () => { const [data, setData] = useState(null); useEffect(() => { fetchData(); }, []); const fetchData = async () => { try { const response = await fetch('https://api.example.com/some-endpoint'); const result = await response.json(); setData(result); } catch (error) { console.error('Error fetching data:', error); } }; return (); }; export default ApiComponent;API Data
{data ? ({data.map((item) => (
) : (- {item.name}
))}Loading...
)}
Step 3: Use the Component in App
Use the ‘ApiComponent’ in your ‘App.js’ or any other component:
// src/App.js import React from 'react'; import ApiComponent from './components/ApiComponent'; function App() { return (); } export default App;
Step 4: Run Your Application
Run your React application:
npm start
Visit http://localhost:3000 in your browser, and you should see your component making an API call and displaying the data.
Using Axios (Optional)
If you prefer using Axios, you need to install it first:
npm install axios
Then, you can modify your ‘ApiComponent.js’:
// src/components/ApiComponent.js import React, { useEffect, useState } from 'react'; import axios from 'axios'; const ApiComponent = () => { const [data, setData] = useState(null); useEffect(() => { fetchData(); }, []); const fetchData = async () => { try { const response = await axios.get('https://api.example.com/some-endpoint'); setData(response.data); } catch (error) { console.error('Error fetching data:', error); } }; return (); }; export default ApiComponent;API Data
{data ? ({data.map((item) => (
) : (- {item.name}
))}Loading...
)}
Both methods, using ‘fetch’ or ‘axios’, are valid, and you can choose the one that fits your preferences or requirements.