Understanding React Hook Form: defaultValues vs values
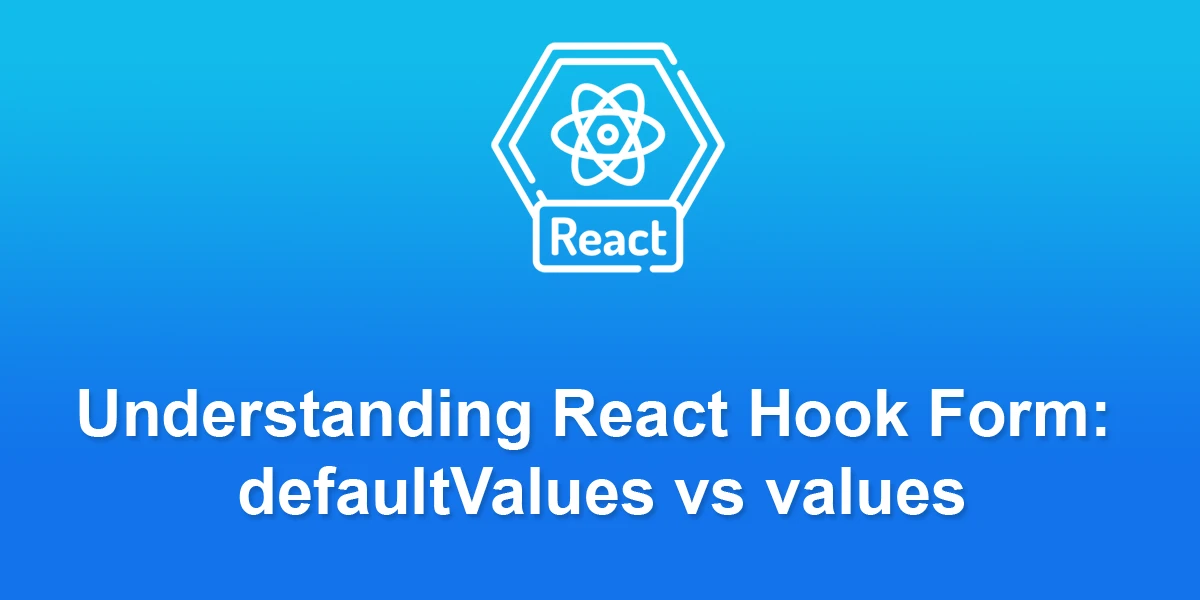
React Hook Form is a popular library for managing forms in React applications. When working with this library, developers often encounter the terms defaultValues and values, which might seem similar at first glance. However, there are key differences between the two that are crucial for effective form management. In this article, we’ll explore these differences and guide you on when to use defaultValues and when to use values.
defaultValues
What are defaultValues?
In React Hook Form, the useForm hook provides the defaultValues option. This option allows you to set initial values for your form fields when the component mounts. These values act as the default values for your form and are useful when you want to prepopulate the form with data.
Example:
import { useForm, Controller } from 'react-hook-form'; function MyForm() { const { control, handleSubmit, setValue } = useForm({ defaultValues: { username: 'JohnDoe', email: 'john@example.com', }, }); const onSubmit = (data) => { // Handle form submission }; return (); }
In this example, the defaultValues option is used to set initial values for the username and email fields.
values
What are values?
While defaultValues is used to set initial values when the form mounts, the values prop is used to dynamically update the form values during its lifecycle. It provides a way to control the values of the form fields programmatically.
Example:
import { useForm } from 'react-hook-form'; function MyForm() { const { register, handleSubmit, setValue, getValues } = useForm(); const setDynamicValues = () => { setValue('username', 'NewUsername'); setValue('email', 'newemail@example.com'); }; const onSubmit = (data) => { // Handle form submission }; return (); }
In this example, the setValue function is used to dynamically update the values of the username and email fields when the “Set Dynamic Values” button is clicked.
When to use defaultValues vs values
- defaultValues: Use defaultValues when you want to set initial values for your form fields when the component mounts. This is suitable for scenarios where the initial values rarely change during the form’s lifecycle.
- values: Use values when you need to dynamically update the form values based on user interactions or other events. This is beneficial for scenarios where the form values need to change during the component’s lifecycle.
In conclusion, understanding the differences between defaultValues and values in React Hook Form is crucial for effective form management. Choose the option that best fits your use case to build dynamic and interactive forms in your React applications.