Navigating the React 16 Warning: “Did Not Expect Server HTML to Contain
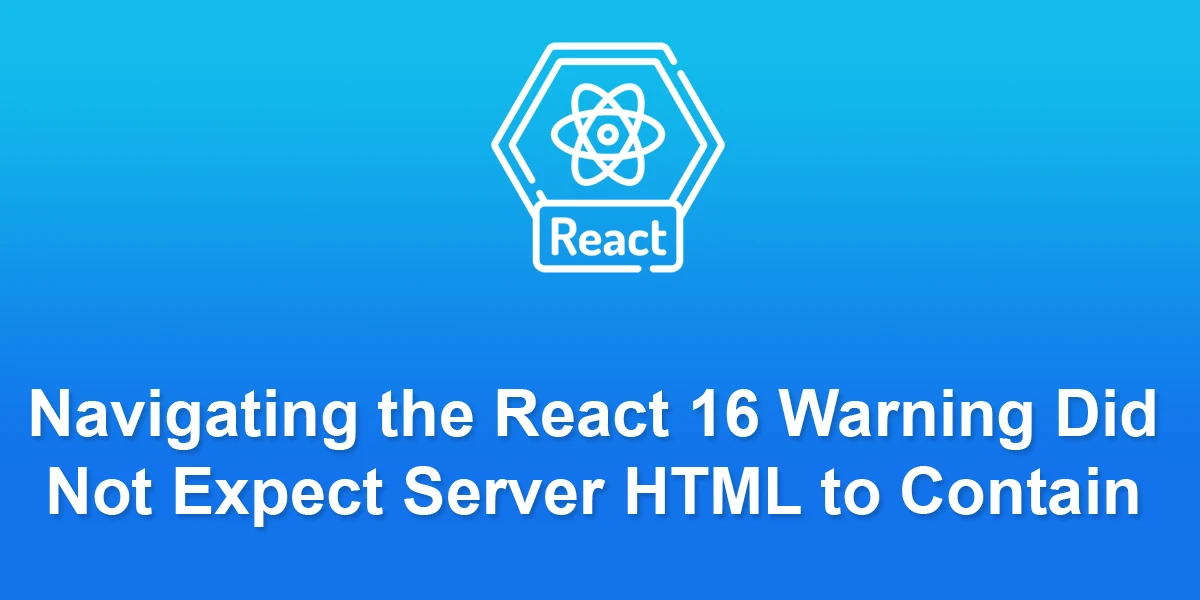
React 16 introduced a more strict server-side rendering (SSR) validation to ensure consistency between the server-rendered HTML and the client-rendered HTML. If you encounter a warning like “Warning: Did not expect server HTML to contain a ‘div’ in ‘div’,” it indicates a mismatch between the server-rendered and client-rendered content. In this article, we’ll explore the causes of this warning and how to address it in your React application.
The warning message suggests that React expected a certain structure when rendering on the server, but the client-rendered HTML did not match those expectations. This can happen when the component tree structure on the server differs from the one on the client, leading to hydration issues.
Common Causes
- 1. Mismatched Component Structure: Check for any differences in the component structure between the server and the client. This can happen if components are conditionally rendered or if the server renders additional elements that the client does not.
- 2. Server-Side Data Fetching: If your components fetch data asynchronously on the server side, ensure that the same data is available on the client side to prevent a mismatch. Consider using techniques like data pre-fetching or passing initial data through the server-rendered HTML.
- 3. Client-Side Manipulation: Avoid manipulating the DOM directly on the client side in a way that modifies the structure generated on the server. React relies on a specific structure for hydration, and deviations can lead to warnings.
Addressing the Warning
- 1. Consistent Component Structure: Ensure that the component structure is consistent between the server and the client. Use conditional rendering consistently and avoid rendering different elements on the server and the client.
- 2. Data Synchronization: If your components fetch data, make sure that the data fetched on the server is available to the client. You can use techniques like passing data through props or incorporating data fetching logic in a way that works seamlessly on both the server and the client.
- 3. Debugging with React DevTools: Use React DevTools to inspect the component tree on both the server-rendered and client-rendered sides. This can help you identify differences in the structure and diagnose the source of the warning more effectively.
- 4. Check Third-Party Libraries: If you’re using third-party libraries that manipulate the DOM or introduce components dynamically, ensure they are SSR-compatible. Some libraries might not play well with server-side rendering, causing hydration issues.
Example Fix
Consider the following example where conditional rendering on the server causes a mismatch:
// Server-Side Rendering const serverRender = isMobile ?: ; const serverHTML = renderToString(serverRender); // Client-Side Rendering const clientRender = isMobile ? : ; ReactDOM.hydrate(clientRender, document.getElementById('root'));
To address this, make sure the rendering logic is consistent:
// Shared Rendering Logic const componentToRender = isMobile ?: ; // Server-Side Rendering const serverHTML = renderToString(componentToRender); // Client-Side Rendering ReactDOM.hydrate(componentToRender, document.getElementById('root'));
The “Did Not Expect Server HTML to Contain a
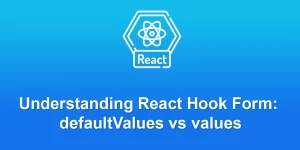
Understanding React Hook Form: defaultValues vs values
March 8, 2024
How to API call in ReactJS step by step guide
February 7, 2024
Create React contact form and send email with EmailJS
February 7, 2024
How to adding facebook login to your React JS project
February 7, 2024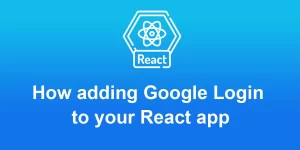
Step by step guide to adding Google login to your React app
February 7, 2024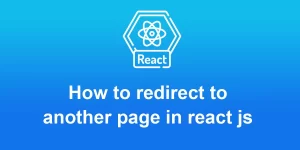
How to redirect to another page in react js step by step
February 6, 2024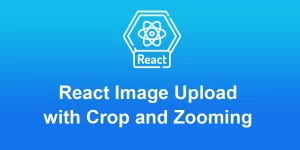
React Image Upload with Crop and Zooming step by step
February 6, 2024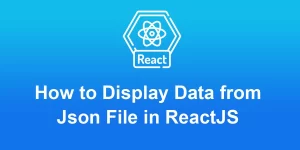
How to Display Data from Json File in react step by step
February 6, 2024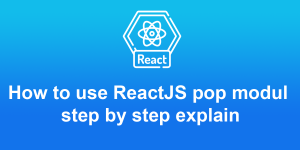
How to use react js pop modul step by step explain
January 31, 2024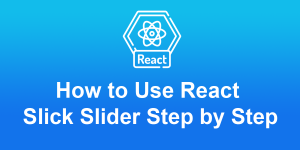