Python and Machine Learning A Practical Guide for Beginners
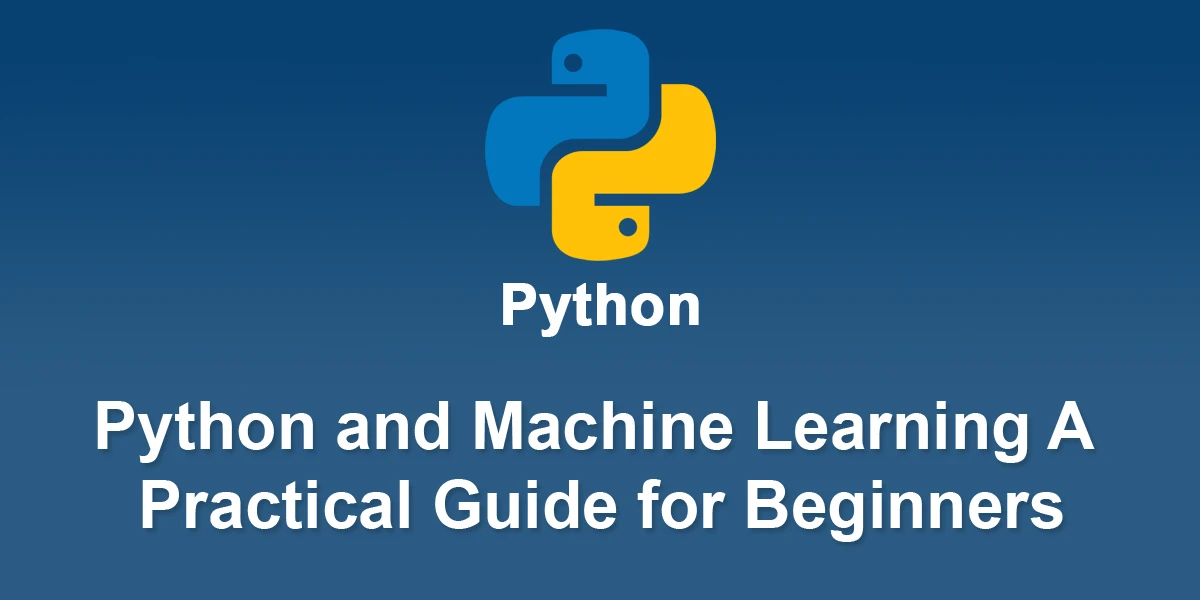
Python is widely used for machine learning due to its simplicity, extensive libraries, and a supportive community. Here’s a practical guide for beginners to get started with Python and machine learning:
1. Setup and Installation:
a. Install Python:
Download and install the latest version of Python from the official Python website.
b. Install Package Manager (pip):
Pip is the package installer for Python. It comes pre-installed with Python 3.4 and above. Ensure it’s available in your system.
2. Install Essential Libraries:
a. NumPy and Pandas:
- NumPy for numerical operations and array handling.
- Pandas for data manipulation and analysis.
pip install numpy pandas
b. Matplotlib and Seaborn:
- Matplotlib for basic plotting.
- Seaborn for statistical data visualization.
pip install matplotlib seaborn
c. Scikit-learn:
Scikit-learn provides simple tools for data mining and data analysis. It includes various machine learning algorithms.
pip install scikit-learn
3. Understand the Basics:
a. Python Basics:
Familiarize yourself with Python basics, such as variables, data types, loops, and functions.
b. NumPy and Pandas Basics:
Learn how to create arrays with NumPy and manipulate data with Pandas.
4. Explore Datasets:
a. Load Sample Datasets:
Use libraries like Seaborn or Scikit-learn to load sample datasets for practice.
import seaborn as sns iris = sns.load_dataset('iris')
5. Build Your First Model:
a. Choose a Simple Model:
Start with a simple machine learning model like linear regression or k-nearest neighbors.
b. Split Data:
Split your dataset into training and testing sets using Scikit-learn.
from sklearn.model_selection import train_test_split X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
c. Train the Model:
Train your model on the training set.
from sklearn.linear_model import LinearRegression model = LinearRegression() model.fit(X_train, y_train)
d. Evaluate the Model:
Use the test set to evaluate the model’s performance.
predictions = model.predict(X_test)
6. Visualize Results:
a. Matplotlib and Seaborn:
Create visualizations to understand your data and model results.
7. Explore Advanced Topics:
a. Feature Engineering:
Learn about feature selection, extraction, and transformation.
b. Cross-Validation:
Understand the importance of cross-validation in evaluating model performance.
c. Hyperparameter Tuning:
Explore techniques for tuning model hyperparameters.
8. Practice with Real-world Data:
a. Kaggle:
Participate in Kaggle competitions or explore datasets to apply your skills on real-world problems.
9. Learn from Resources:
a. Online Courses and Tutorials:
Platforms like Coursera, edX, and Udacity offer excellent machine learning courses.
b. Books:
Consider reading books like “Hands-On Machine Learning with Scikit-Learn, Keras, and TensorFlow” by Aurélien Géron.
10. Join the Community:
a. Forums and Communities:
Engage with the Python and machine learning communities on platforms like Stack Overflow and Reddit.
11. Experiment and Iterate:
- Try Different Models:
- Experiment with various machine learning algorithms to understand their strengths and weaknesses.
- Iterate and Improve:
- Iterate on your models based on feedback and performance evaluation.
Remember, machine learning is an iterative process, and continuous learning and experimentation are key to mastering it. Start with simple projects, gradually tackle more complex problems, and build your expertise over time.