Python Web Development From Basics to Deployment
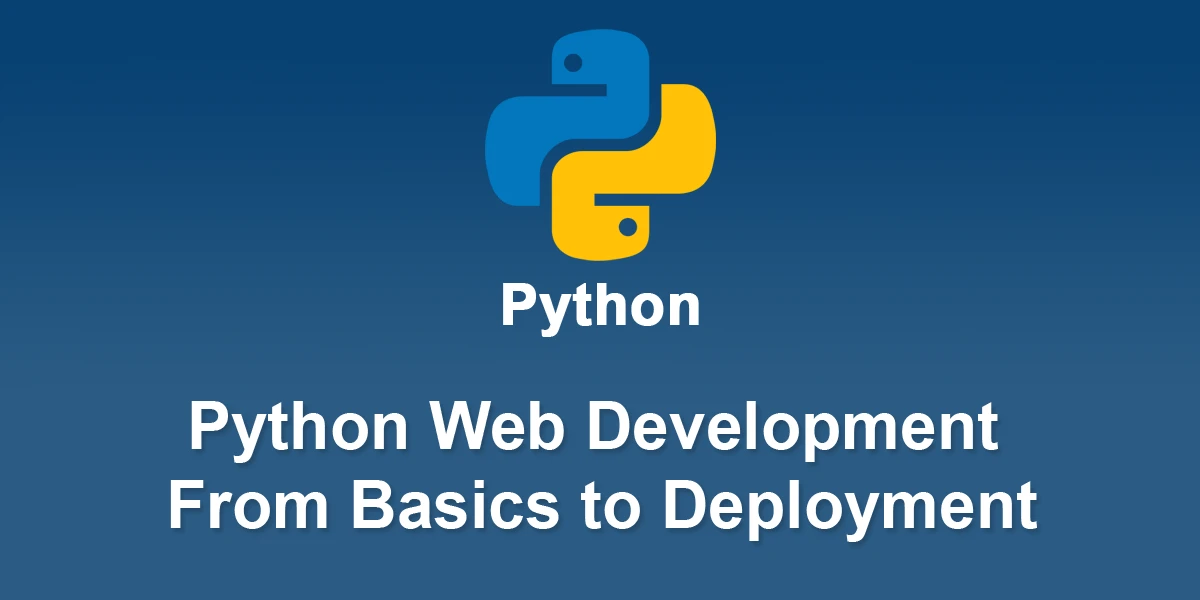
Here’s a step-by-step guide for Python web development, from basics to deployment, using Flask as the web framework
Step 1: Setup and Installation
1. Install Python:
Download and install the latest version of Python from python.org.
1. Install Virtual Environment:
Open a terminal or command prompt and create a virtual environment for your project.
python -m venv myenv
3. Activate Virtual Environment:
Activate the virtual environment.
On Windows:
myenv\Scripts\activate
On macOS/Linux:
source myenv/bin/activate
Step 2: Install Flask
1. Install Flask:
Within the activated virtual environment, install Flask using pip.
pip install Flask
Step 3: Create a Simple Flask App
1. Create Project Folder:
Create a new folder for your project.
mkdir myflaskapp cd myflaskapp
2. Create App File:
Create a file named app.py and add the following code.
from flask import Flask app = Flask(__name__) @app.route('/') def hello(): return 'Hello, Flask!'
3. Run the App:
Run your Flask app.
python app.py
Open your browser and go to http://127.0.0.1:5000/ to see your Flask app in action.
Step 4: HTML Templates and Static Files
1. Create Templates Folder:
Inside your project folder, create a folder named ‘templates’.
mkdir templates
2. Create HTML Template:
Create an HTML file inside the ‘templates’ folder.
My Flask App Hello, Flask!
3. Update Flask App:
Modify your app.py to render the HTML template.
from flask import Flask, render_template app = Flask(__name__) @app.route('/') def hello(): return render_template('index.html')
Step 5: Deployment with Heroku
1. Create ‘requirements.txt’:
Create a ‘requirements.txt’ file listing your project dependencies.
pip freeze > requirements.txt
2. Create Procfile:
Create a ‘Procfile’ (without any file extension) in your project folder with the following content:
web: python app.py
3. Initialize Git Repository:
Initialize a Git repository in your project folder.
git init
1. Commit Changes:
Add and commit your changes.
git add . git commit -m "Initial commit"
5. Heroku Setup:
- Install the Heroku CLI from here.
- Login to your Heroku account.
heroku login
6. Create a Heroku App:
Create a new Heroku app.
heroku create
7. Deploy to Heroku:
Deploy your app to Heroku.
git push heroku master
8. Open the App:
Open your deployed app in the browser.
heroku open
Congratulations! You’ve successfully created a simple Flask app and deployed it to Heroku. This guide covers the basics, and you can continue to explore more advanced features and topics as you delve deeper into Python web development.