Web Scraping with Python A Practical Guide
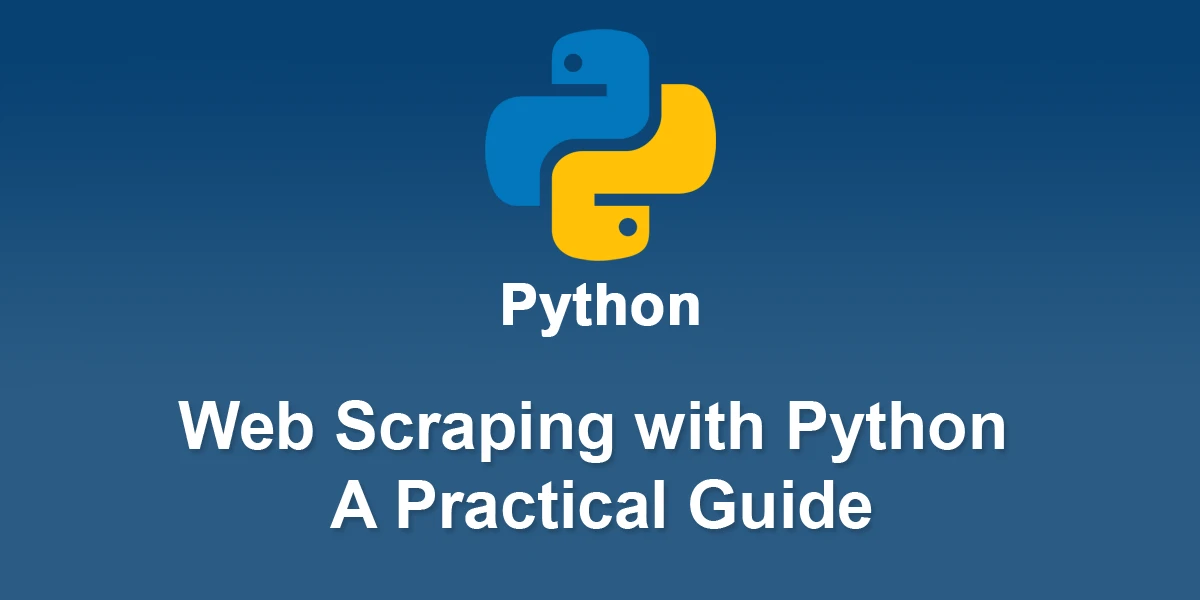
Web scraping is the process of extracting data from websites, and Python provides powerful libraries like BeautifulSoup and requests for this purpose. Here’s a practical guide to web scraping with Python:
1. Install Required Libraries:
pip install requests beautifulsoup4
2. Understand HTML Basics:
Familiarize yourself with HTML structure, tags, and elements. This knowledge will help you locate and extract data from web pages.
3. Inspect Web Page:
Use your web browser’s developer tools (right-click and select “Inspect” or press Ctrl+Shift+I) to inspect the HTML structure of the web page you want to scrape. Identify the HTML elements containing the data you need.
4. Use ‘requests’ to Fetch HTML:
import requests url = 'https://example.com' response = requests.get(url) html = response.text
5. Parse HTML with BeautifulSoup:
from bs4 import BeautifulSoup soup = BeautifulSoup(html, 'html.parser')
6. Locate Data:
Use BeautifulSoup to navigate the HTML and locate the specific elements containing the data you want to scrape. Methods like ‘find()’, ”, and CSS selectors can be helpful.
# Example using CSS selector titles = soup.select('.title-class')
7. Extract Data:
Extract the desired data from the located HTML elements. Depending on the structure of the data, you may need to use text extraction methods or access specific attributes.
for title in titles: print(title.text)
8. Handling Pagination:
If the data spans multiple pages, understand how pagination works and implement a solution to navigate through pages.
9. Dealing with Dynamic Content:
For websites that load content dynamically using JavaScript, you may need to use tools like Selenium along with a webdriver.
10. Save Data:
Save the scraped data to a file (e.g., CSV, JSON, or database). Libraries like pandas can be useful for handling and storing structured data.
import pandas as pd df = pd.DataFrame({'Title': titles}) df.to_csv('scraped_data.csv', index=False)
11. Respect Robots.txt:
Check the website’s robots.txt file to ensure you are not violating any terms of service. Always respect the website’s policies on web scraping.
12. Handling Errors:
Implement error handling to deal with potential issues like network errors, missing elements, or changes in website structure.
13. Legal and Ethical Considerations:
Ensure that your web scraping activities comply with legal and ethical standards. Do not scrape sensitive or personal information without proper authorization.
14. Use User Agents:
Some websites may block requests from bots. Set a user agent to mimic requests from a web browser.
headers = {'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36'} response = requests.get(url, headers=headers)
15. Rate Limiting:
Avoid making too many requests in a short period to prevent being blocked by the website. Implement rate limiting by adding delays between requests.
import time time.sleep(2) # Pause for 2 seconds
16. Explore API Options:
Check if the website provides an API for accessing data. Using an API may be more efficient and ethical than web scraping.
17. Keep Abreast of Changes:
Websites may update their structure, requiring adjustments to your web scraping code. Monitor the website and update your code accordingly.
npm start
18. Experiment and Test:
Experiment with different scenarios, test your code on various websites, and refine your web scraping skills by building small projects.
Web scraping can be a powerful tool, but it’s essential to use it responsibly and ethically. Always check a website’s terms of service and ensure your scraping activities comply with legal and ethical standards.