Canvas Color Magic: Changing the Background Color Dynamically
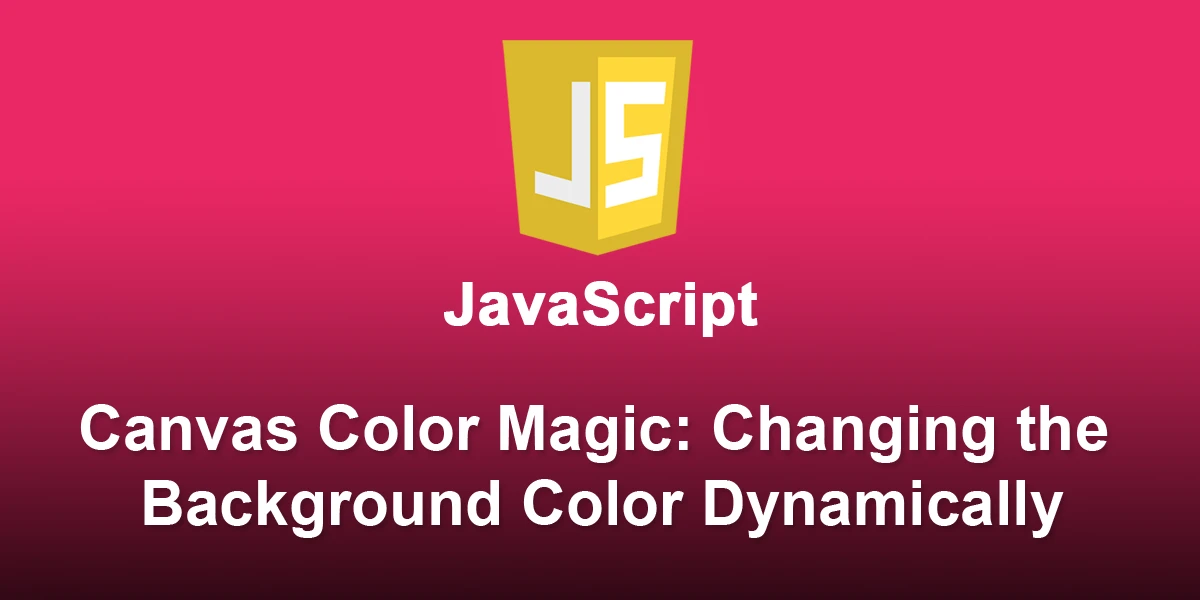
The HTML canvas element serves as a blank slate for creating dynamic graphics and visualizations on the web. Changing the background color of a canvas is a fundamental task in web development, and it’s crucial for customizing the appearance of your canvas-based projects. In this article, we’ll explore the simple yet impactful process of dynamically changing the background color of a canvas using JavaScript.
Setting Up the Canvas
Before diving into the code, ensure you have an HTML file with a
Canvas Background Color
In this example, we have a canvas element with an id of “myCanvas” and dimensions set to 400×200 pixels. The JavaScript code will be placed in the “script.js” file.
Changing the Background Color
To change the background color of the canvas dynamically, we’ll use the getContext method to obtain the canvas rendering context and set the fillStyle property.
// script.js // Get the canvas element and its 2D context const canvas = document.getElementById('myCanvas'); const context = canvas.getContext('2d'); // Set the new background color context.fillStyle = 'lightblue'; // Fill the entire canvas with the new color context.fillRect(0, 0, canvas.width, canvas.height);
In this code snippet:
- We obtain the canvas element and its 2D rendering context.
- The fillStyle property is set to the desired color (‘lightblue’ in this case).
- The fillRect method is used to draw a filled rectangle covering the entire canvas, effectively changing the background color.
Making it Interactive
You can enhance the user experience by making the canvas background color change on user interaction. Let’s modify the code to change the background color when the user clicks on the canvas:
// script.js // Get the canvas element and its 2D context const canvas = document.getElementById('myCanvas'); const context = canvas.getContext('2d'); // Add a click event listener to the canvas canvas.addEventListener('click', changeBackgroundColor); // Function to change the background color function changeBackgroundColor() { // Generate a random color using RGB values const randomColor = `rgb(${Math.random() * 255}, ${Math.random() * 255}, ${Math.random() * 255})`; // Set the new background color context.fillStyle = randomColor; // Fill the entire canvas with the new color context.fillRect(0, 0, canvas.width, canvas.height); }
Now, every time the user clicks on the canvas, the background color will change to a random color.
Changing the background color of a canvas is a simple yet impactful way to customize the visual appeal of your web projects. Whether you choose a static color or make it interactive, these techniques provide the flexibility to create engaging and dynamic user interfaces.