Google Places Autocomplete Location Search Example in JavaScript
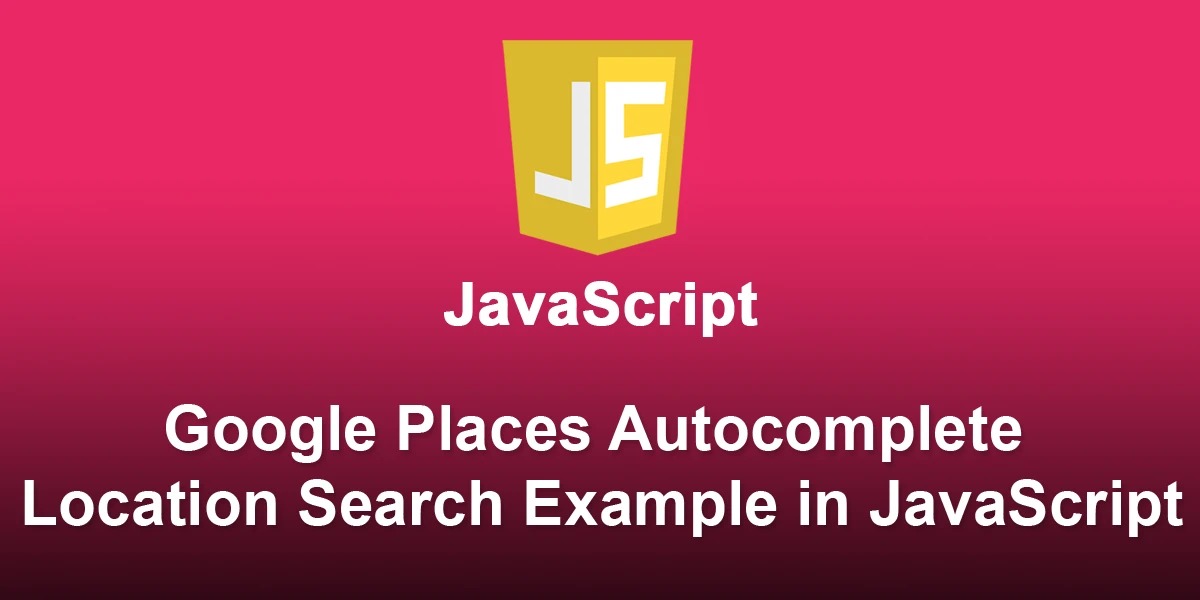
Google Places Autocomplete is a powerful feature that enhances the user experience when dealing with location inputs on web applications. In this tutorial, we’ll explore how to implement Google Places Autocomplete using JavaScript, providing users with real-time location suggestions as they type.
Prerequisites: Before we begin, make sure you have obtained a Google Places API key from the Google Cloud Console. This key is essential for accessing the Google Places Autocomplete API.
Step 1: Include Google Places Autocomplete API Script
Include the Google Places Autocomplete API script in your HTML file.
Google Places Autocomplete Example
Replace YOUR_API_KEY with your actual Google Places API key.
Step 2: Implement JavaScript for Autocomplete
Create a JavaScript file (script.js) to handle the Google Places Autocomplete functionality.
// script.js document.addEventListener("DOMContentLoaded", function() { // Initialize the autocomplete with options const autocomplete = new google.maps.places.Autocomplete(document.getElementById('autocomplete'), { types: ['geocode'], }); // Add an event listener for place changed event autocomplete.addListener('place_changed', function() { const place = autocomplete.getPlace(); // Log place details to console (you can customize this based on your needs) console.log('Place Name:', place.name); console.log('Place Address:', place.formatted_address); console.log('Place ID:', place.place_id); console.log('Place Geometry:', place.geometry.location); }); });
Step 3: Test the Application
- Open the HTML file in a web browser.
- Start typing in the input field, and you’ll see real-time location suggestions.
- Select a suggestion from the dropdown, and the selected place details will be logged to the console.
Customizing the Autocomplete
You can customize the autocomplete behavior and appearance by adjusting the options provided during initialization. Explore the Google Places Autocomplete API documentation for detailed information on available options.
Implementing Google Places Autocomplete in your web application using JavaScript significantly improves the user experience when dealing with location inputs. Users can easily find and select locations, and developers gain access to rich place details.