Drag and Drop Image Display with HTML and JavaScript
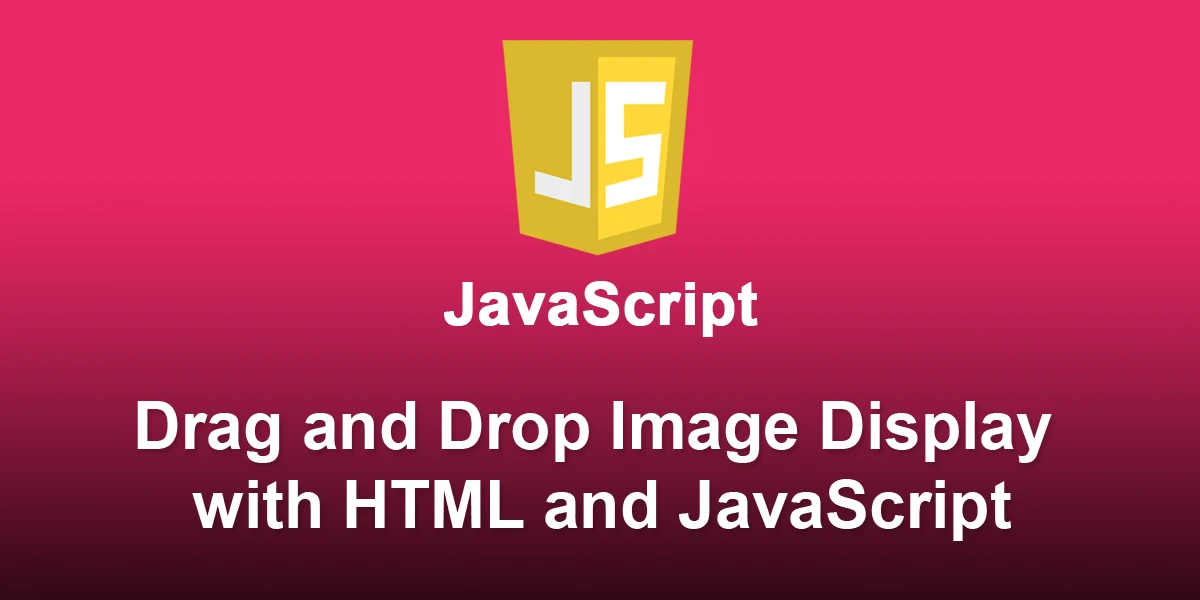
Enhancing user interaction on your web page is always a plus, and one engaging way to do this is by enabling users to drag and drop images directly onto the page. In this tutorial, we’ll guide you through the process of creating a simple HTML page where users can drag and drop an image, and the page dynamically displays the dropped image using JavaScript.
Step 1: Setting Up the HTML Structure
Let’s start by setting up the basic HTML structure. Create an HTML file and add the following content:
Drag and Drop Image Display Drag & Drop an Image Here
![]()
Step 2: Styling with CSS
Now, let’s add some basic styling to make the drop container visually appealing. Create a styles.css file:
body { display: flex; justify-content: center; align-items: center; height: 100vh; margin: 0; } .drop-container { border: 2px dashed #ccc; padding: 20px; text-align: center; cursor: pointer; } img { max-width: 100%; max-height: 80vh; display: none; }
Step 3: JavaScript Magic
The real magic happens in the JavaScript file. Create a script.js file:
const dropArea = document.getElementById('dropArea'); const displayedImage = document.getElementById('displayedImage'); // Prevent default drag behaviors ['dragenter', 'dragover', 'dragleave', 'drop'].forEach(eventName => { dropArea.addEventListener(eventName, preventDefaults, false); document.body.addEventListener(eventName, preventDefaults, false); }); // Highlight drop area when item is dragged over it ['dragenter', 'dragover'].forEach(eventName => { dropArea.addEventListener(eventName, highlight, false); }); // Remove highlight when item is dragged out of drop area ['dragleave', 'drop'].forEach(eventName => { dropArea.addEventListener(eventName, unhighlight, false); }); // Handle dropped files dropArea.addEventListener('drop', handleDrop, false); function preventDefaults(e) { e.preventDefault(); e.stopPropagation(); } function highlight() { dropArea.classList.add('highlight'); } function unhighlight() { dropArea.classList.remove('highlight'); } function handleDrop(e) { const dt = e.dataTransfer; const files = dt.files; if (files.length > 0) { const reader = new FileReader(); reader.onload = function (e) { displayedImage.src = e.target.result; displayedImage.style.display = 'block'; }; reader.readAsDataURL(files[0]); } }
Step 4: Test and Customize
Save your files, open the HTML file in a browser, and test the drag and drop functionality. Feel free to customize the styles or add additional features to suit your project.
Conclusion
Congratulations! You’ve successfully created a dynamic image display using drag and drop functionality with HTML and JavaScript. This feature adds a touch of interactivity to your web page, providing a user-friendly and engaging experience.