From Monday to Sunday A Locale-Based Week Start Detection With JavaScript
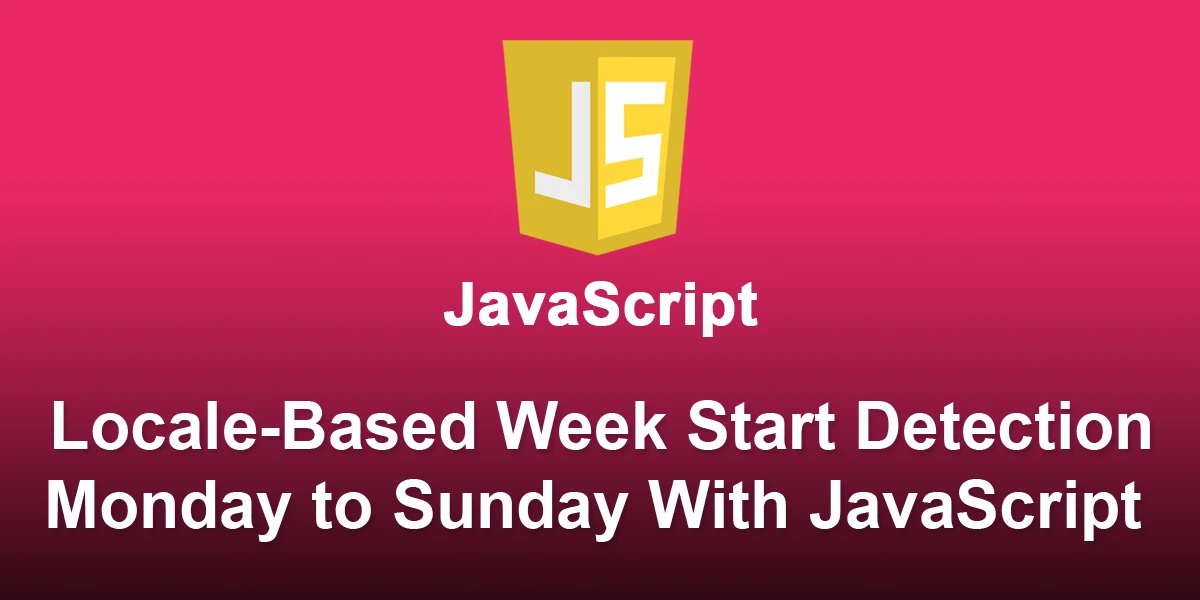
When working with date-related operations in JavaScript, understanding the local conventions for the start of the week is crucial. Some locales consider Monday as the first day of the week, while others adhere to Sunday. In this guide, we’ll explore how to determine the start day dynamically based on locale using pure JavaScript.
Understanding Locale-Based Week Start
Different regions and cultures follow distinct conventions regarding the start of the week. For example, in the United States, Sunday is often considered the first day, while in many European countries, Monday holds that honor.
To accommodate these differences in your JavaScript applications, you can create a function that dynamically determines the start day of the week based on the user’s locale.
The Code Breakdown
Let’s dive into a simple yet effective JavaScript function that accomplishes this task:
function getWeekStartDay() { const locale = navigator.language || 'en-US'; // Get user's locale or default to 'en-US' const dayIndex = new Date(1970, 0, 4).toLocaleDateString(locale, { weekday: 'short' }); // Check if the locale starts the week with Sunday or Monday return dayIndex === 'Sun' ? 'Sunday' : 'Monday'; } // Example Usage const startDay = getWeekStartDay(); console.log(`The week starts on ${startDay}.`);
How It Works
- Get the User’s Locale: The navigator.language property is used to obtain the user’s locale. If unavailable, it defaults to ‘en-US’ (English, United States).
- Determine the Day Index: We create a Date object set to January 4, 1970, which was a Sunday. By formatting this date using toLocaleDateString and specifying { weekday: ‘short’ }, we retrieve the abbreviated day name (e.g., ‘Sun’ or ‘Mon’).
- Check Locale Convention: The obtained day index is then checked against ‘Sun’. If it matches, the week starts on Sunday; otherwise, it starts on Monday.
- Example Usage: Finally, we demonstrate how to use the function and print the result.
Conclusion
By dynamically determining the start day of the week based on the user’s locale, your JavaScript applications can seamlessly adapt to various cultural preferences. This approach ensures a more user-friendly experience, particularly in internationalized applications where date and time representations play a crucial role.