Preventing Swiping Between Web Pages in iPad Safari Using JavaScript
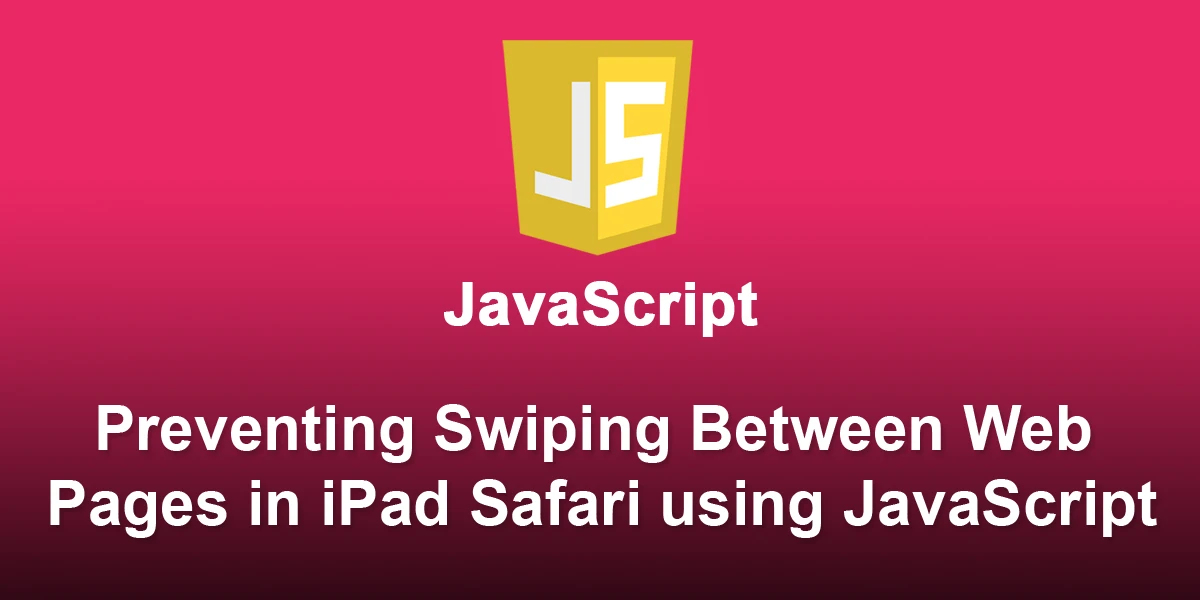
Introduction
iPad Safari provides a smooth and intuitive browsing experience, complete with gestures like swiping between web pages. However, there are scenarios where preventing this behavior becomes necessary, such as when building single-page applications (SPAs) or interactive web experiences that require a controlled flow. In this article, we’ll explore how to prevent swiping between web pages on iPad Safari and enhance user interaction.
The Challenge
By default, iPad Safari enables users to navigate between previously visited pages by swiping left or right. This can be undesired behavior in certain web applications where a custom navigation flow is implemented. To overcome this, we can use a combination of JavaScript and CSS to disable the swiping gesture.
Implementation
1. CSS Styles
Start by adding the following CSS styles to your project. These styles will help to disable the swipe gesture globally:
html, body { overflow-x: hidden; overscroll-behavior-y: contain; touch-action: pan-right pan-left; }
Explanation:
- overflow-x: hidden;: Prevents horizontal scrolling.
- overscroll-behavior-y: contain;: Disables the overscroll effect on the vertical axis.
- touch-action: pan-right pan-left;: Specifies that the touch gesture should only trigger panning to the right or left.
2. JavaScript
Now, include the following JavaScript code in your project to handle touch events and prevent swiping:
document.addEventListener('touchstart', handleTouchStart, false); let xStart = null; function handleTouchStart(event) { const firstTouch = event.touches[0]; xStart = firstTouch.clientX; } document.addEventListener('touchmove', function(event) { if (xStart !== null) { const xDiff = xStart - event.touches[0].clientX; if (xDiff > 5 || xDiff < -5) { event.preventDefault(); } xStart = null; } }, false);
Explanation:
- touchstart: Captures the initial touch position.
- touchmove: Prevents the default behavior if the horizontal movement exceeds a certain threshold, effectively disabling the swipe gesture.
Integration
Include the CSS styles and JavaScript code in your project. Ensure that the styles are applied globally, and the JavaScript is executed when the page loads.
Your Web App
Conclusion
By combining CSS styles and JavaScript, you can successfully prevent swiping between web pages in iPad Safari, providing a more controlled and seamless user experience in scenarios where custom navigation is crucial. Ensure to test thoroughly on different devices to guarantee compatibility and usability.